- 책 대출하기
2023.12.16 - [Spring/[PROJECT] 도서 대여 프로그램] - 💛 책 대출 등록하기 💛
💛 책 대출 등록하기 💛
Controller → Service → Repository 🔖 Controller ☑️ 책의 대출 정보가 담긴 데이터 (addRentDto) 가 들어온다 ☑️ 책이 이미 대출 중인지, 대출 가능한 상태인지 확인한다 (BookStatus) ☑️ 대출이 가능하면
alsrudalsrudalsrud.tistory.com
📕 Controller
☑️ 요청이 오면, DB 에 저장되어 있는 책 대출 목록을 반환한다 - service 메서드 호출 (getAllRent)
...
public class RentController {
private final RentService rentService;
// 책 대출 목록 조회
@GetMapping()
public List<ResponseRentListDto> getAllRent() {
return rentService.getAllRent();
}
...
}
📕 Service
☑️ Repository 의 findAll 메서드로 모든 책 대출 정보를 가져온다
☑️ 원하는 정보만 반환하기 위해 Dto 를 사용한다 (ResponseRentListDto)
...
public class RentService {
private final RentRepository rentRepository;
private final MemberRepository memberRepository;
private final BookRepository bookRepository;
// 책 대출 목록 조회
public List<ResponseRentListDto> getAllRent() {
List<Rent> rentList = rentRepository.findAll();
return rentList.stream()
.map(ResponseRentListDto::responseRentList)
.collect(Collectors.toList());
}
...
}
📕 Repository
☑️ findAll 메서드를 사용하기 위해, JpaRepository 인터페이스를 상속받는다
...
public interface RentRepository extends JpaRepository<Rent, Long> {}
📕 Dto
☑️ 원하는 정보만을 반환하는 데이터를 담는다
@Getter
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class ResponseRentListDto {
private String memberName;
private String bookName;
public static ResponseRentListDto responseRentList(Rent rent) {
ResponseRentListDto rentListDto = ResponseRentListDto.builder()
.memberName(rent.getMember().getNickname())
.bookName(rent.getBook().getTitle())
.build();
return rentListDto;
}
}
💡TEST💡
· Postman
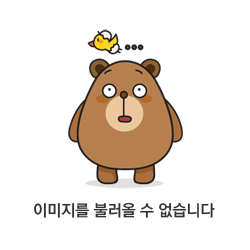
'Spring > [P] 도서 대여 프로그램' 카테고리의 다른 글
🩷 좋아요 기능 (추가, 취소) 🩷 (0) | 2023.12.16 |
---|---|
💛 책 반납하기 💛 (0) | 2023.12.16 |
💛 책 대출 등록하기 💛 (0) | 2023.12.16 |
💚 등록된 책 조회하기 (전체 조회, 선택 조회) 💚 (0) | 2023.12.16 |
💚 책 등록하기 💚 (0) | 2023.12.12 |