- Controller → Service → Repository
🔖 Controller
☑️ 책의 대출 정보가 담긴 데이터 (addRentDto) 가 들어온다
☑️ 책이 이미 대출 중인지, 대출 가능한 상태인지 확인한다 (BookStatus)
☑️ 대출이 가능하면 Rent 테이블에 등록한다 - service 메서드 호출 (createRent)
@RestController
@RequestMapping("/rent")
@RequiredArgsConstructor
public class RentController {
private final RentService rentService;
// 책 대출 등록
@PostMapping()
public ResponseEntity<String> createRent(@RequestBody AddRentDto addRentDto) {
// 1. BookStatus 확인 후 변경 : AVAILABLE -> UNAVAILABLE
HttpStatus bookStatus = rentService.checkBookStatus(addRentDto);
// 2. Rent table 등록
if (bookStatus == HttpStatus.CONFLICT) {
return new ResponseEntity<>("이미 대출 중인 책입니다", HttpStatus.CONFLICT);
} else {
rentService.createRent(addRentDto);
return new ResponseEntity<>("책을 대출하였습니다", HttpStatus.OK);
}
}
}
🔖 Service
☑️ createRent 메서드 : addRentDto 를 통해 입력된 회원 id, 책 id 를 통해 각각의 객체를 조회한 후, DB 에 대출 정보를 저장한다
☑️ checkBookStatus 메서드 : 해당 책의 대출 상태가 AVAILABLE 인지 UNAVAILABLE 인지 확인 후 HttpStatus를 반환한다
@Service
@Transactional
@RequiredArgsConstructor
public class RentService {
private final RentRepository rentRepository;
private final MemberRepository memberRepository;
private final BookRepository bookRepository;
// 책 대출 등록
public void createRent(AddRentDto addRentDto) {
Member member = memberRepository.findById(addRentDto.getMemberId())
.orElseThrow(RuntimeException::new);
Book book = bookRepository.findById(addRentDto.getBookId())
.orElseThrow(RuntimeException::new);
rentRepository.save(new Rent(member, book));
}
// 책 상태 변경 (AVAILABLE -> UNAVAILABLE)
public HttpStatus checkBookStatus(AddRentDto addRentDto) {
Book book = bookRepository.findById(addRentDto.getBookId()).orElseThrow(RuntimeException::new);
// 이미 대출 중인 책일 경우 (BookStatus : UNAVAILABLE)
if (book.getBookStatus() == BookStatus.UNAVAILABLE) {
return HttpStatus.CONFLICT;
} else {
// 대출 가능한 책일 경우 (BookStatus : AVAILABLE -> UNAVAILABLE)
book.changeBookStatus(BookStatus.UNAVAILABLE);
return HttpStatus.OK;
}
}
}
🔖 Repository
☑️ DB 에 저장하는 save 메서드를 사용하기 위해 JpaRepository 인터페이스를 상속받는다
@Repository
public interface RentRepository extends JpaRepository<Rent, Long> {}
🔖 Dto
☑️ 책의 대출 정보를 담은 데이터를 담게 한다 - 회원 ID, 책 ID
@Getter
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class AddRentDto {
private Long memberId;
private Long bookId;
public static AddRentDto addRent(Rent rent) {
AddRentDto rentDto = AddRentDto.builder()
.memberId(rent.getMember().getId())
.bookId(rent.getBook().getId())
.build();
return rentDto;
}
}
💡TEST💡
· Postman
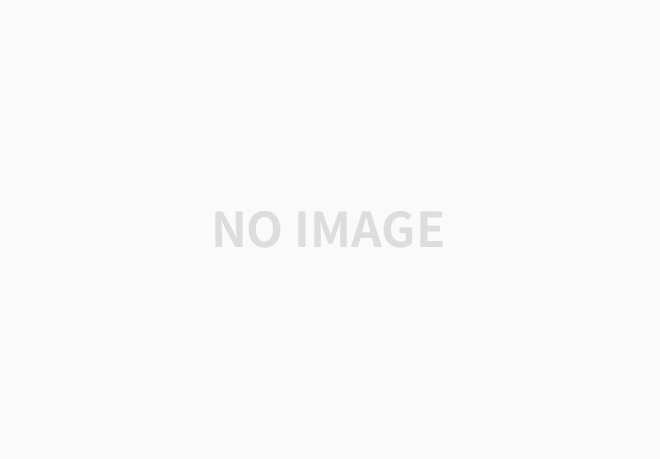
· Datagrip
☑️ Rent Table 에 등록된 것 확인하기
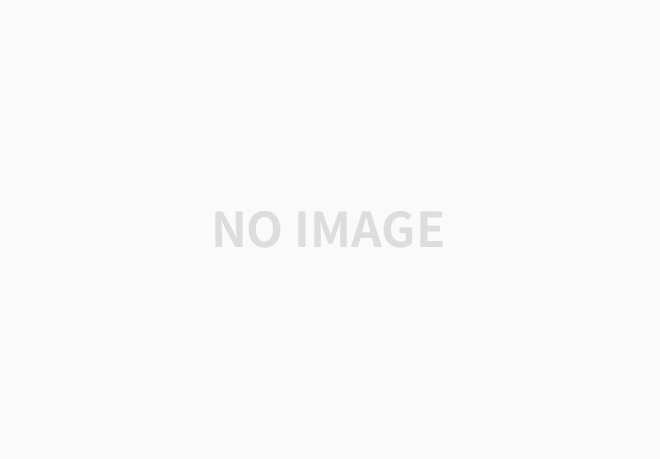
☑️ 빌린 책의 bookStatus 변경된 것 확인하기
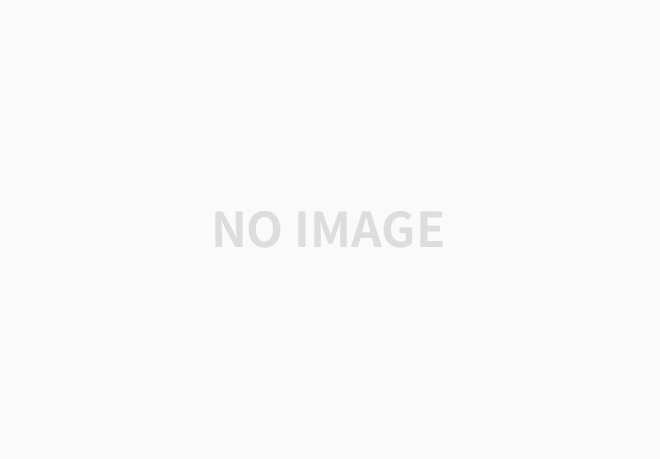
'Spring > [P] 도서 대여 프로그램' 카테고리의 다른 글
💛 책 반납하기 💛 (0) | 2023.12.16 |
---|---|
💛 대출한 책 조회하기 💛 (0) | 2023.12.16 |
💚 등록된 책 조회하기 (전체 조회, 선택 조회) 💚 (0) | 2023.12.16 |
💚 책 등록하기 💚 (0) | 2023.12.12 |
🩵 Spring Security (2) 로그인 🩵 (0) | 2023.11.28 |